When learning software development and programming languages, you are introduced to different programming paradigms, such as Procedural, Object-Oriented (OOP), Imperative, Declarative, and more. Each paradigm has a different purpose and is used to classify certain programming languages.
This article is about the Functional Programming paradigm – one of software development's most essential and extensively used programming structures.
Here is a sneak peek of what you will learn upon further reading:
- What is the Functional Programming paradigm?
- Why Functional Programming?
- Pros and cons of Functional Programming
- Popular Functional Programming languages
Ready to take a roller coaster ride into the world of Functional Programming? Let's go!
What is the Functional Programming Paradigm?
A programming paradigm that encourages program development to be done purely with functions is called the Functional Programming paradigm (FP). In this structure, the developer writes code in pure mathematical expressions. It is designed to handle symbolic computation and application processing.
This approach treats functions as first-class citizens, meaning that functions can be:
- Stored in variables
- Passed to other functions as arguments
- Returned by other functions
By adopting this programming approach, you can write clean and maintainable code. It mainly focuses on "What to solve" rather than "How to solve."
Characteristics of Functional Programming
The following features of Functional Programming make it a viable paradigm. Let's discuss each in detail:
Pure Functions
Pure functions always return the same value for the same input. They don't modify any external state or have any observable actions apart from returning a value. This behavior of providing predictable return value without causing any hidden changes to program state results in better clarity of the code and more efficient debugging and testing.
Example:
First-class Functions
The first class functions are the ones that you can treat as a variable, i.e., you can manipulate them just like variables. You can pass them to and return from other functions. The key benefit of these functions is that there are no limitations over the usage of functions.
High-order Functions
When a function takes a function as an argument or returns a function, it is known as a higher-order function. These functions are foundational in Functional Programming, allowing for powerful modularisation and abstraction. They allow patterns such as map, reduce, and filter, which can operate on data structures in a polymorphic manner.
Immutability
All data is immutable in Functional Programming, meaning no existing value state will change. If an argument is passed to a function, the state of the argument is not changed. Instead, it returns a new value, leaving the original one unchanged.
Declarative Approach
Functional Programming adopts the declarative approach, meaning its main focus is "What" rather than "How."
Lazy Evaluation
Lazy evaluation means an expression is not evaluated unless its value is needed, i.e., a function that contains or requires it is not called. This approach reduces unintended side effects and makes the behavior of functions more predictable.
Recursion
One of the main goals of Functional Programming is to avoid the use of flow control structures (loops, break, goto, continue, etc.). The best way to achieve this is recursion.
Recursion is when a function calls itself repeatedly unless it meets an exit condition. A developer may use this structure instead of loops to avoid complexity and increase the system's maintainability.
Example:
Why Functional Programming?
Although Functional Programming has existed since the 1930s, it has gained much popularity recently due to the rise of machine learning and big data.
This paradigm is a good choice when you need to analyze large data sets in a short time. Moreover, its modular structure and fast error detection also enable you to more efficiently debug your code.
Pros and Cons of Functional Programming
Just like any other programming paradigm, Functional Programming has its perks and drawbacks.
Pros:
- Modular Structure: FP allows you to break down larger software into small programs based on features called modules. Each module represents a particular feature, making the code simple and easy to comprehend.
- Error Prevention: By supporting stateless programming, the FP allows you to avoid unintentional changes in data, making it very easy to test and debug your code.
- Parallel Programming: Due to immutability, Functional Programming makes it easier to run different modules in parallel without any chance of the side effects of mutable data. This increases the testability and reusability of your programs.
- Concurrency: The stateless function in FP can reduce the complexity of concurrency, reducing the change of race conditions and deadlocks. However, concurrency issues can still arise, especially if the underlying system or libraries introduce shared state or other side effects.
- Lambda Calculus Implementation: Using Functional Programming, you can implement lambda calculus to solve complex problems in your programs.
Cons:
- More Memory Consumption: Some features of functional programming, such as recursive functions, can consume considerable memory space, especially in languages that lack tail call optimisation. Also, immutability in functional programming means that instead of modifying existing data structures, new ones are often created, which can further increase memory usage. The Discord team overcame this by using Rust.
- State Management: Real-world applications often involve a lot of state management, and while there are functional ways to manage state (e.g., Monads in Haskell), they can be difficult to grasp and can complicate code.
- Difficult to Learn: Learning Functional Programming is quite challenging, especially for beginners, as it originates from Lambda calculus, which handles computing as mathematics.
- Less Mainstream Adoption: Functional Programming, while gaining traction, is still less popular than the OOP paradigm. This can result in fewer resources, libraries, and community support available for developers. Also, finding experienced FP developers for team projects can be more challenging compared to those proficient in OOP.
Popular Functional Programming Languages
There are many signs that show Functional Programming is trending and gaining popularity among software developers. Here's a short list of popular FP languages that you can learn to excel in the field:
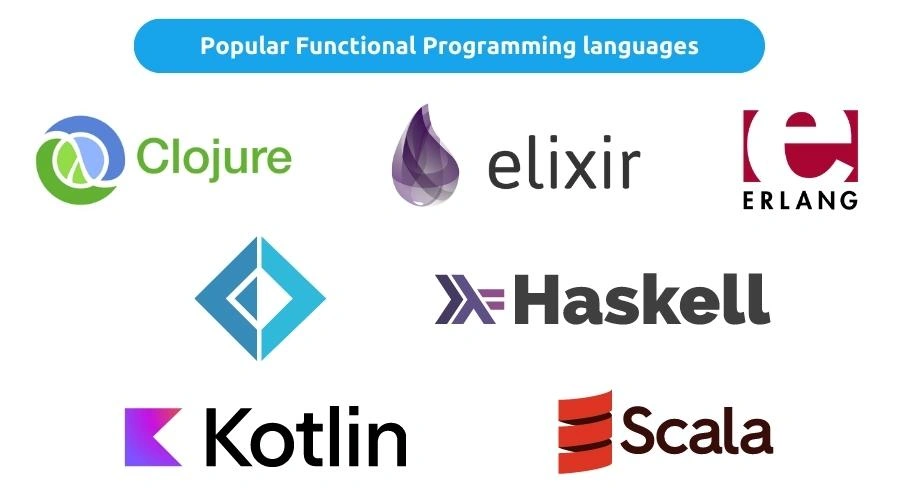
- Clojure
- Elixir
- Erlang
- F#
- Haskell
- Kotlin
- Scala (both OOP and functional)
Final thoughts
The Functional Programming paradigm is an excellent approach to achieving maintainable, testable, and sustainable programs.
However, FP also has its drawbacks, such as potentially higher memory consumption, complex state management, and a steep learning curve.
In this article, we have discussed Functional Programming, its features, pros and cons, and uses. We have also suggested a few Functional Programming languages you can learn to excel in your software development career.
We hope you've learned something new. See you on the next one!